Make Zip File Java !!
How to create a zip file in Java - Stack Overflow
Creating a zip file with single file in it / Adding single file to an existing zip new ZipFile("filename.zip").addFile("filename.ext"); Or new ZipFile("filename.zip").addFile(new File("filename.ext")); I have a dynamic text file that picks content from a database according to the user's query. I have to write this content into a text file and zip it in a folder in a servlet. How should I do this?This will create a zip in the root of D: named test.zip which will contain one single file called mytext.txt. Of course you can add more zip entries and also specify a subdirectory like this:
To write a ZIP file, you use a ZipOutputStream. For each entry that you want to place into the ZIP file, you create a ZipEntry object. You pass the file name to the ZipEntry constructor; it sets the other parameters such as file date and decompression method. You can override these settings if you like. Then, you call the putNextEntry method of the ZipOutputStream to begin writing a new file. Send the file data to the ZIP stream. When you are done, call closeEntry. Repeat for all the files you want to store. Here is a code skeleton:
Here is an example code to compress a Whole Directory(including sub files and sub directories), it's using the walk file tree feature of Java NIO.
Given exportPath and queryResults as String variables, the following block creates a results.zip file under exportPath and writes the content of queryResults to a results.txt file inside the zip.
You have mainly to create two functions. First is writeToZipFile() and second is createZipfileForOutPut . and then call the createZipfileForOutPut('file name of .zip')` â¦
I know this question is answered but if you have a list of strings and you want to create a separate file for each string in the archive, you can use the snippet below.
If you want decompress without software better use this code. Other code with pdf files sends error on manually decompress
Since it took me a while to figure it out, I thought it would be helpful to post my solution using Java 7+ ZipFileSystem
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.23.39825
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.

How to create Zip file in Java - Mkyong.com
In Java, we can use `java.util.zip` and `Files.copy` to zip a single file or a whole directory. Java 7 introduced the Zip File System Provider, combines with Files.copy, we can copy the file attributes into the zip file easily (see example 4).2.1 This example uses the Java 7 NIO FileSystems.newFileSystem to create a zip file and Files.copy to copy the files into the zip path.
This example uses ByteArrayInputStream to directly create some bytes on demand and save it into the zip file without saving or writing the data into the local file system.
4.2 This Java example uses FileVisitor to walk a file tree and ZipOutputStream to zip everything manually, including sub-files and subdirectories, but ignore symbolic links and file attributes.
The above example creates the zip file at the current working directory, and we didn’t copy the file attributes (review the file created date and time).
5.1 This example uses the same FileVisitor to walk the file tree. Still, this time we use FileSystems URI to create the zip file, and Files.copy to copy the files into the zip path, including the file attributes, but ignore the symbolic link.
The zip4j is a popular zip library in Java; it has many advanced features like a password-protected zip file, split zip file, AES encryption, etc. Please visit the zip4j github for further documentation and usages.
Is there a way to create multiple txt files (without creating the txt files locally) which will be zipped and then send back to the user as a zipped folder? I have created a web service which enables the user to download a zipped folder with specific txt files. But in order to do that, I have to create the txt files locally -> zip them -> delete the txt files from the local storage.
When the service is deployed, I am not allowed to create files on the server. I saw that some people mentioned to create the files in-memory but there are risks. There is a case that I might run out of memory.
The above method is used to create and return a File. I have a List which contains all the txt files. I iterate the list and add the files into ZipOutputStream etc.
I managed to find a solution using the combination of example 3 and my code. The website does not allow me to delete my old comments in order to upload the code.
The code on example 3 did not work for me when I used Postman to send a GET request (selected Send and Download).
“great” example…Sry, but this example creates invalid ZIP files. Within ZIP files you should ALWAYS use forward slashes for paths – this is described within the ZIP standard (https://pkware.cachefly.net/webdocs/casestudies/APPNOTE.TXT). this example creates backward slashes for paths on windows file systems. I wouldn’t blame you, if you were using unix, but obviously you ARE using windows. Please adapt this to avoid others creating invalid ZIPs.
I have a requirement to zip an XML file generated in the same code flow. I am marshalling a java object to outputstream. But I don’t want to write the XML file. Because all I need is the zip file, XML file is not being used later.
I was trying to discover if we can directly zip the outputstream of xml into a zip file without creating the intermediate XML file.
My last option would be, creating the XML file, then creating the ZIP file from it and then deleting the XML file.
Hi did you get the code? if you can you please share the code i am also facing the same problem please hep.
Hi mkyong thank you for this example 5.Zip a folder or directory -FileSystemsIt’s working fine on linux but not on windows.
Hello Mkyong, thanks for the example. Just one improvement: … FileOutputStream fos = new FileOutputStream(zipFile); BufferedOutputStream bos = new BufferedOutputStream(fos); ZipOutputStream zos = new ZipOutputStream(bos); … Wrapping the FileOutputStream into a BufferedOutputStream will make zip-file creation MUCH faster.
Hi Kong, I tested this code , In last line “SOURCE_FOLDER.length()+1 ” , +1 is not required. Am I right?
Hi, thank you a lot, But It Has A Problem!!! if we get it an empty folder, it will throw exception, and also, if we have an empty folder in another folder, it doesn’t create that empty folder!
Hello Sir, I have a requirement where client can download all uploaded file from server. I have done downloading for single file. But i am not able to allow user to download all file at a single click Please Help me with this. all files are image file and i am suppose to zip it and allow download to user pc. Thank you Praveen
Hi mkyong thank you for this section it is interesting but the code does not work it gives an empty archive 🙁
If i have a list of files, and i would like to rename it beofre zip it up, how could that be done?Can anyone advise? Many Thanks.
The design for the blog is a tad off in Epiphany. Nevertheless I like your website. I may need to use a normal web browser just to enjoy it.
Hi Yong, it’s necessary to considered that compressing directories which are empty in the example of ‘Advance ZIP example – Recursively’. thank you for sharing,I like the platform.
You can use Java mail to create an e-mail, attach that zip file and send it to that designated address.
Mkyong.com is providing Java and Spring tutorials and code snippets since 2008. All published articles are simple and easy to understand and well tested in our development environment.
How to create a zip file in Java - Programmer Gate
How to create a zip file in Java. by Hussein Terek · July 27, 2019. Fatal error: Uncaught Error: Call to a member function id () on array in /home/customer/www/programmergate.com/public_html/wp-content/plugins/crayon-syntax-highlighter/crayon_formatter.class.php:36 Stack trace: #0 /home/customer/www/programmergate. by Hussein Terek · July 27, 2019
Create .zip File in Java using Apache Commons Compress
Create New .zip File from a Directory. In the following example Java program, we use the above class to create a new file file. In the example we have “D:\SimpleSolution\sample.zip” is the .zip file that is expected to be created. “D:\SimpleSolution\sample\” is the directory that needs to be zip. CreateZipFileFromDirectoryExample.java In this Java tutorial, we learn how to use the Apache Commons Compress library to create a .zip file in the Java program.To use Apache Commons Compress Java library in the Gradle build project, add the following dependency into the build.gradle file.
To use Apache Commons Compress Java library in the Maven build project, add the following dependency into the pom.xml file.
First step, we implement a new class named ZipFileCompressUtils and introduce createZipFile() public method to create a .zip file from a file or directory source.
In the following example Java program, we use the above class to create a new file file. In the example we have
How to create ZIP file inside a ZIP file in Java - Stack Overflow
Create inner zip file like this: ByteArrayOutputStream innerZipBufferOutput = new ByteArrayOutputStream(BUFFER_SIZE); ZipOutputStream innerZipOutput = new ZipOutputStream( new BufferedOutputStream(innerZipBufferOutput)); Write bytes (info) to the inner zip I am trying to create a ZIP file inside a ZIP file to re-construct a previously in memory zip structure I got in Java.I am failing since I get an error on the inner ZIP created inside the initial ZIP file. The file is corrupted. I get an "unexpected ending of file" when trying to open it.
The Code unzip it all in memory using a java Stack and a Map. Then it creates input2.zip, with innerInput.zip in it.
Summary: I need to create a ZIP with a ZIP in it, all in memory (not saving on disk temporarily).
Ok, the problem is storing a ZIP within a ZIP right?Well, I was getting an error because the inner zip was closed too soon
Create inner zip file like this: ByteArrayOutputStream innerZipBufferOutput = new ByteArrayOutputStream(BUFFER_SIZE); ZipOutputStream innerZipOutput = new ZipOutputStream( new BufferedOutputStream(innerZipBufferOutput));
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.23.39825
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
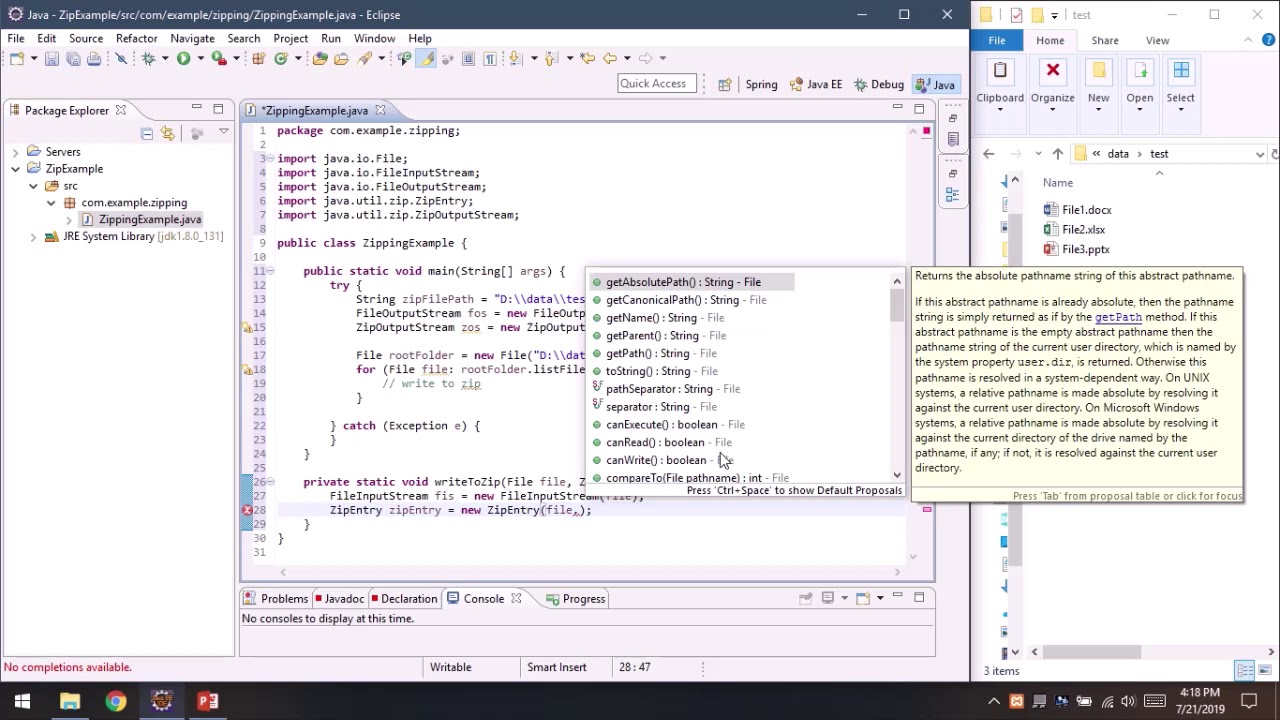
Creating zip archive in Java - Stack Overflow
// simplified code for zip creation in java import java.io.*; import java.util.zip.*; public class ZipCreateExample { public static void main(String[] args) throws Exception { // input file FileInputStream in = new FileInputStream("F:/sometxt.txt"); // out put file ZipOutputStream out = new ZipOutputStream(new FileOutputStream("F:/tmp.zip")); // name the file inside the zip file out.putNextEntry(new ZipEntry("zippedjava.txt")); // buffer size byte[] b = new byte[1024]; int count; while I have one file created by 7zip program. I used deflate method to compress it. Now I want to create the same archive (with the same MD5sum) in java. When I create zip file, I used the algorithm that I found on the Internet for example http://www.kodejava.org/examples/119.html but when I created zip file with this method the compressed size is higher than size of the uncompressed file so what is going on? This isn't a very useful compression. So how I can create zip file that is exactly same as zip file that I created with 7zip program ? If it helps I have all information about zip file that I created in 7zip program.Just to clarify, you used the ZIP algorithm in 7zip for your original? Also 7zip claims to have a 2-10% better compression ratio than other vendors. I would venture a guess that the ZIP algorithm built into Java is not nearly as optimized as the one in 7zip. Your best best is to invoke 7zip from the command line if you want a similarly compressed file.
Are you trying to unpack a ZIP file, change a file within it, then re-compress it so that it has the same MD5 hash? Hashes are meant to prevent you from doing that.
Sets the default compression method for subsequent entries. This default will be used whenever the compression method is not specified for an individual ZIP file entry, and is initially set to DEFLATED.
Sets the compression level for subsequent entries which are DEFLATED. The default setting is DEFAULT_COMPRESSION. level - the compression level (0-9)
Here is a function that you pass the absolute path it will create a zip file with the same name as the directory (under which you want zip of all the sub folder and files, everything !!) and return true on success and false on exception if any.
To generate two identical zip files (including identical md5sum) from the same source file, I would recommend using the same zip utility -- either always use the same Java program, or always use 7zip.
The 7zip utility for instance has a lot of options -- many of which are simply defaults that can be customized (or differ between releases?) -- and any Java zip implementation would have to also set these options explicitly. If your Java app can simply invoke an external "7z" program, you'll probably get better performance anyway that a custom Java zip implementation. (This is also a good example of a map-reduce problem where you can easily scale out the implementation.)
But the main issue you will run into if you have a server-side generated zip file and a client-side generated zip file is that the zip file stores two things in addition to just the original file: (1) the file name, and (2) the file timestamp. If either of these have changed, then the resulting zip file will have a different md5sum:
You'll find the same problem with differing filenames and paths, even when the files inside the zip are identical.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.23.39825
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
java - Create a Zip File in Memory - Stack Overflow
Use ByteArrayOutputStream with ZipOutputStream to accomplish the task. you can use ZipEntry to specify the files to be included into the zip file. Here is an example of using the above classes, String s = "hello world"; ByteArrayOutputStream baos = new ByteArrayOutputStream (); try (ZipOutputStream zos = new ZipOutputStream (baos)) { /* File isHow to compress files in ZIP in Java - JavaPointers
Running this code will create the zip file in our project directory: Decompressing ZIP in Java To decompress a zip file, we just need to use the ZipInputStream in Java and iterate each ZipEntry and save it to our destination folder.Zipping and Unzipping in Java | Baeldung
In this quick tutorial, we'll discuss how to zip a file into an archive and how to unzip the archive – all using core libraries provided by Java. These core libraries are part of the java.util.zip package – where we can find all zipping and unzipping related utilities. 2. Zip a Filecompression - How can I create multipart compressed zip file in java
ZipFile.createZipFile(File sourceFile, ZipParameters parameters, boolean splitArchive, long splitLength) is the method to create a split zip file. boolean splitArchive has to be set to true in this case. You can set the maximum file size for each split file (z01, z02, etc) via long splitLength make zip file javajava make zip file from directory
java code to make zip file
make zip file using java
javascript make zip file
java make zip file from files
make zip file java
make a wish nct,make a wish nct lyrics,make a wish,make a wish drama,make a wish nct lyrics english,make a wish chinese drama,make a google account,make a wish artinya,make a gif,make america great again,zip adalah,zip artinya,zip apk,zip archiver,zip app,zip android,zip archive php,zip a dee doo dah,zip asx,zip atau rar,file adalah,file ai adalah,file a report twitter,file artinya,file ai,file an appeal twitter,file anime,file autocad,file apk,file audio,java adalah,java array,java aquatic,java arraylist,java abstract class,java api,java adventure company,java apple,java applet,java android